How to list assets using keywords and Server Side JavaScript (SSJS)
There are several ways to list assets in Matrix, including menu design areas, asset listings, and search pages.
These are quite powerful and have lots of configurable options that are available to them.
Sometimes though, you might want to have a quicker and more code-based way of listing some assets. For example, you might be working on a page template that only needs to list the child assets of a page. Or you may have a related asset metadata field from which you need to list assets.
Using keywords and SSJS and keywords, you can achieve quick asset lists using code and avoid extra assets and configuration screens.
In this how-to, you use SSJS and keywords inside a paint layout to dynamically list child image assets under the page to which the layout is applied.
Before you start
-
You have some basic knowledge of using JavaScript and have read and understood the basic concept of Server Side JavaScript.
Create an asset structure
Before you start adding any code, you need a basic asset structure in place.
To get the structure described in this section, you can Download tutorial assets used in this tutorial and import them into your Matrix system using the Import Assets from XML tool. |
-
Create a Standard page asset named "Page".
-
Create a Paint Layout asset and apply the paint layout to the "Page" asset.
-
Below the Standard page asset, create six Image assets using any images you like.
The structure you create with the steps above should look similar to this image:
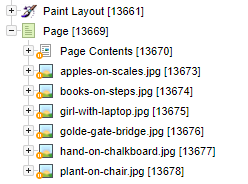
Add the keyword
The only place we’ll be adding code is in the Default Format of the Paint Layout. So go ahead and open up the Edit Contents screen of that asset.
The first thing you need is to get a list of all the assets to print in a format suitable for JavaScript.
-
Open the Edit Contents view of the Paint Layout asset and find the Default Content section.
-
Add the
%asset_children_link_type_2%
keyword under the%asset_contents%
keyword already in the Paint Layout.<div> %asset_contents% </div> %asset_children_link_type_2% (1)
1 This keyword returns an array of asset IDs of all the child assets directly below the standard page. The _link_type_2
part of the keyword tells it only to bring backHidden link
linked children. -
Select Save.
-
Preview the page on the frontend.
Notice that you have a Heading and an unformatted array of asset IDs corresponding to the Image assets you created in the Before you start section. The keyword is only bringing back the asset ID of each child asset. However, for a complete listing, you also need to get the name, URL, and asset type code for the image listing.
To do that, we can use the ^as_asset
keyword modifier to specify what other values we want to retrieve as part of this keyword.
-
In the Default Content section add the
^as_asset:asset_name,asset_type_code,asset_url
keyword modifier to the%asset_children_link_type_2%
keyword.<div> %asset_contents% </div> %asset_children_link_type_2^as_asset:asset_name,asset_type_code,asset_url% (1)
1 You can add as many standard asset fields, attributes, or metadata values to this chain as you need. A comma must separate each value. If you are retrieving asset IDs from a Related Asset Metadata Field value, you must use the ^json_decode
keyword modifier as well. For example,%asset_metadata_relatedjson_decodeas_asset:asset_name,asset_type_code,asset_url%
. -
Select Save
If you preview the page on the frontend at this point, notice that the keyword is returning a JSON object array for the image assets you created in the Before you start section. While the data is there, it is not formatted very nicely.
Wrap the keyword in a <script> tag and send it to the browser’s console for easier inspection:
-
Locate the Default Content section.
-
Wrap the keyword in
<script>
tags.<div> %asset_contents% </div> <script> console.log(%asset_children_link_type_2^as_asset:asset_name,asset_type_code,asset_url%); </script>
-
Select Save
-
Preview the page in a new tab and open your browser’s developer tools console.
You can see the benefits of wrapping the keyword with
<script>
tags, so the data is formatted cleanly.
Now that you have the right data coming through, you can work on the presentation layer: the Server Side JavaScript (SSJS).
Code the listing using SSJS
In the same code block, you can now start writing some SSJS code around the keyword that prints images on the frontend.
-
In the Default Content section, put the keyword value into a variable.
<script runat="server"> //Get all of our assets into an array variable const assets = %asset_children_link_type_2^as_asset:asset_name,asset_type_code,asset_url^empty:[]%; (1) </script>
1 The ^empty:[]
keyword modifier at the end of the keyword is a fallback just in case the variable returns an empty list of children. -
Wrap an
if
statement around the variable. Only loop over the results if there are any items returned in the list.<script runat="server"> //Get all of our assets into an array variable const assets = %asset_children_link_type_2^as_asset:asset_name,asset_type_code,asset_url^empty:[]%; //Only print if there are at least 2 child assets if(assets.length > 1){ (1) //Loop through each asset in the array assets.forEach(function(asset){ print(asset); }); } </script>
1 Notice that the code checks if the length of the array is greater than 1
rather than0
. Because the asset type is a standard page, the child list always includes the Page Contents Bodycopy directly below the asset (the first array position:0
). Therefore, if there is at least one image under the page, the array length is always greater than1
. -
Add formatting to the SSJS to make the items appear in a list with some validation added:
<script runat="server"> //Get all of our assets into an array variable const assets = %asset_children_link_type_2^as_asset:asset_name,asset_type_code,asset_url^empty:[]%; //Only print if there are at least 2 child assets if(assets.length > 1){ print('<ul>'); (1) //Loop through each asset in the array assets.forEach(function(asset){ //Only print if it's an image asset if(asset.asset_type_code === 'image'){ (2) print('<li><img src="'+ asset.asset_url +'" title="'+ asset.asset_name +'" width="100"/></li>'); } }); print('</ul>'); } </script>
1 Enclose the keyword object as a <li>
tag in a<ul>
tag that prints only if the child assets returned are greater than1
.2 To make sure that each object listed is an image asset, add an if
statement to check if theasset_type_code
value is equal to'image'
for each item printed. -
Select Save and preview the page to view the final result.
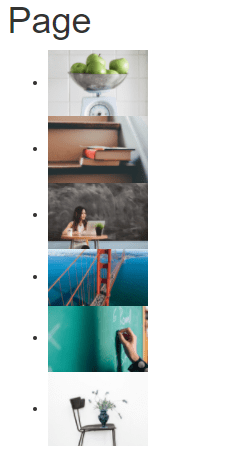
Summary
With just a single keyword and a few lines of JavaScript, you have quickly created a code-based asset listing.
You could also extend this keyword to include metadata value from each asset.
To retrieve all metadata values of an asset, you can use the asset_data_metadata
keyword to keep the keyword you see in this example shorter and more readable.