REST API (V2)
Choose the right component
Use the flowchart below to assist in choosing the appropriate component for your use case:
The example below shows the development team creation using the REST API component with Squiz Connect’s own REST API service.
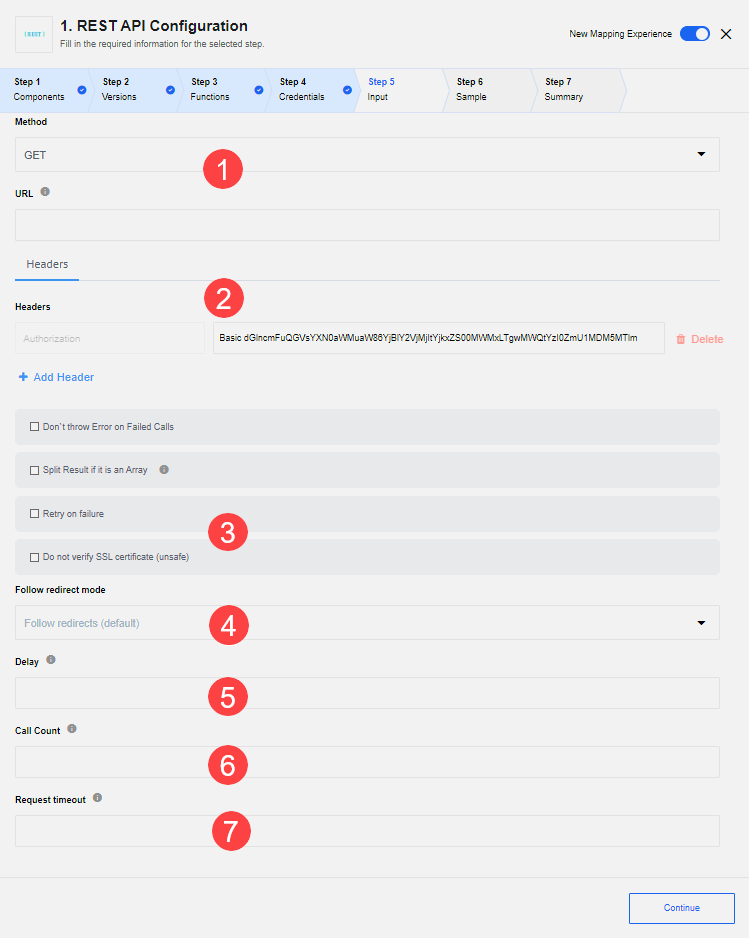
-
The Method and URL of the REST API resource:
-
REST API component supports the following HTTP methods:
GET
,PUT
,POST
,DELETE
, andPATCH
. -
The URL of the REST API resources. Accepts JSONata expressions - i.e. the URL address evaluates JSONata expressions.
-
-
Headers - specify the request headers and body:
-
Configuration options:
- Don’t throw Error on Failed Calls
-
If enabled, return an error, an error code, and a stack trace in the message body otherwise, throw an error in flow.
- Split Result if it is an Array
-
If enabled and response is an array, create a message for each item of the array. Otherwise, create one message with response array.
- Retry on failure
-
Enabling rebound feature for following HTTP status codes:
-
408: Request Timeout
-
423: Locked
-
429: Too Many Requests
-
500: Internal Server Error
-
502: Bad Gateway
-
503: Service Unavailable
-
504: Gateway Timeout
-
DNS lookup timeout
-
- Do not verify SSL certificate (unsafe)
-
Disable verifying the server certificate - unsafe.
-
Follow redirect mode - Choose either the option Follow redirects (default) or Do not follow redirects to perform either of these functions.
-
Delay - Set a delay value here (in seconds) to slow down requests to your API. This content type delays calling the subsequent request by this specified number of seconds after the previous request.
The time for the delay is calculated as Delay/Call Count and should not be more than 1140 seconds (19 minutes due to preset platform limits). The Call Count default value is 1. If you want to use another value, set the Call Count field.
Learn more about the Delay value in Known limitations. -
Call count - This field’s value (whose default value is 1) is only used in combination with the Delay field.
-
Request timeout - The timeout period in milliseconds (1-1140000) that the REST API component waits for the server to respond. The default value of this field is 100000 (100 sec). If the configuration field is not provided, this field’s behavior can also be configured with the
REQUEST_TIMEOUT
environment.If both the REQUEST_TIMEOUT
environment variable and the Request timeout field value are specified for this component, then this field value takes precedence.
Authorization methods
To use the REST API component with any restricted access API, you must provide the authorization information.
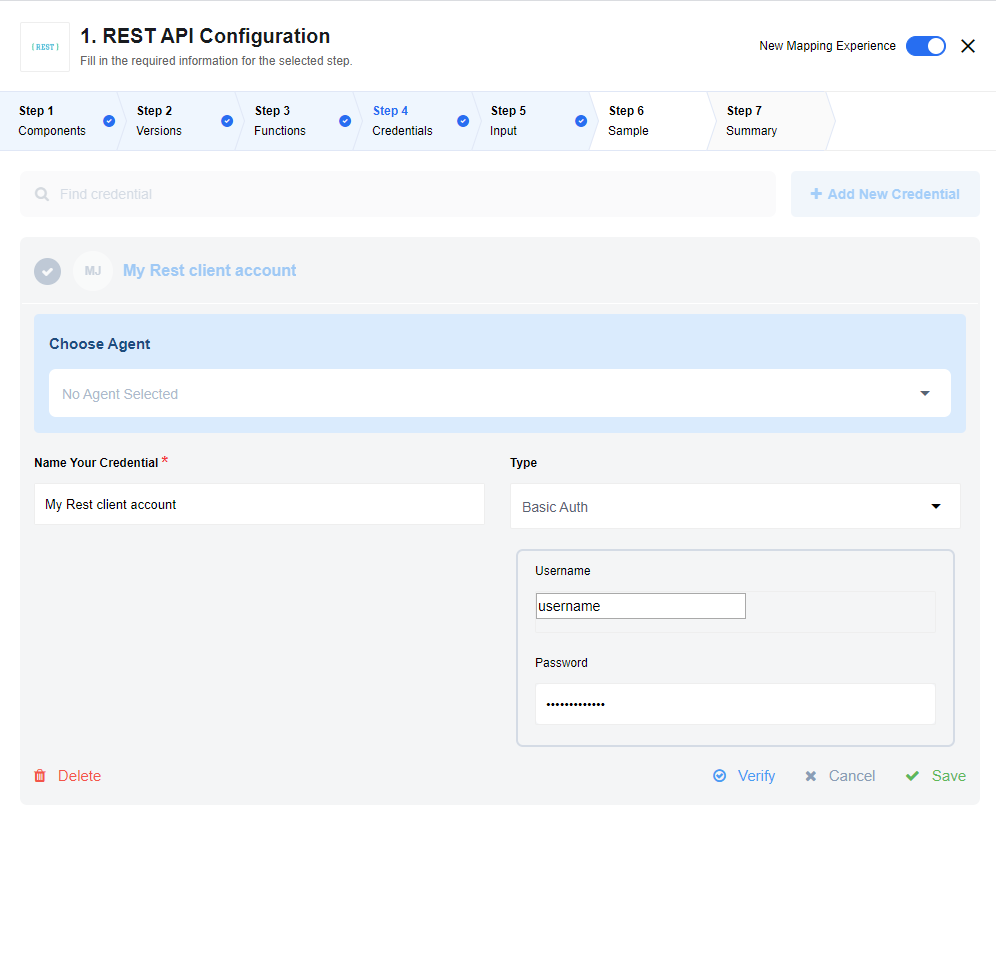
This example shows how to add the username/password to access the API during the integration flow design.
You can add the authorization methods during the integration flow design or by navigating to your
from the main menu and adding the methods there.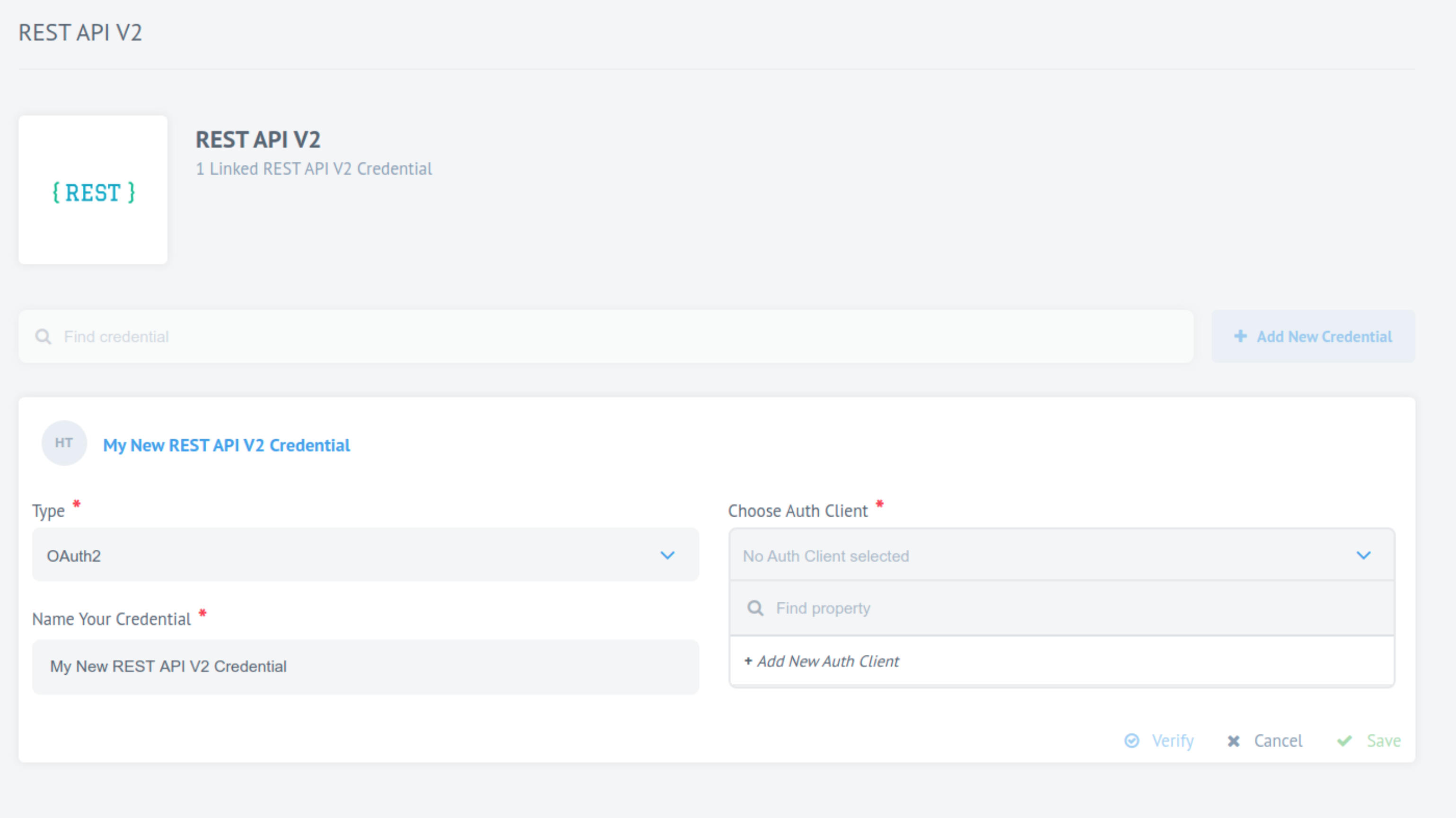
This example above shows how to add a new credential to access the API from the credentials page.
REST API component supports the following authorization types:
- No Auth
-
Use this method to work with any open REST API. This method can be used without the need for verification.
- Basic Auth
-
Use this method to provide sign-in credentials like username/password.
- API Key Auth
-
Use this method for systems where an API Key is required to access the resource. This authorization type requires a Header Name (for example,
api-key
) and its accompanying Header Value (for example, the value ofapi-key
) values. - OAuth2
-
Use this method when the external resource dictates an
Oauth2
authorization to access their resources.To create an OAuth2 credential, you have to choose Auth-client or create the new one. This credential must contain Name, Client ID, Client Secret, Authorization Endpoint and Token Endpoint. Read the documentation of the API to which you want to connect for more information.
This example shows how to add a new Auth-client to access the API.
The following example shows you how to select an existing client:
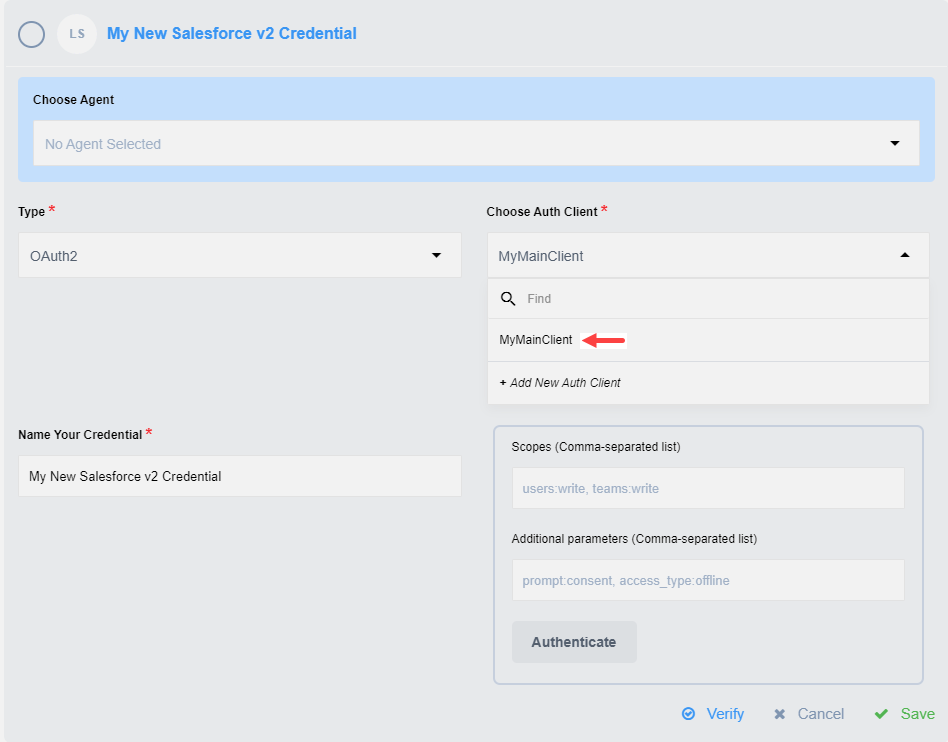
Environment variables
NAME | DESCRIPTION | DEFAULT | OPTIONAL |
---|---|---|---|
REQUEST_TIMEOUT |
HTTP authorization request timeout in milliseconds. |
10000 |
true |
REQUEST_RETRY_DELAY |
Delay between authorization retry attempts in milliseconds |
5000 |
true |
REQUEST_MAX_RETRY |
Number of HTTP authorization request retry attempts. |
3 |
true |
Triggers and actions
The REST API component can be used as either a trigger or an action.
A cron schedule is configured as a trigger, as is the case for the simple trigger component.
As an action, this component sends an HTTP request using a GET
/POST
/PUT
/PATCH
/DELETE
method and parses the response back to the flow.
Output
The messages produced by the REST API component have the following properties:
- headers
-
Object containing the HTTP response headers.
- statusCode
-
HTTP Status Code of the Response. This code is a number between
100
and599
. - statusMessage
-
Human readable equivalent to the response code.
- body
-
The contents of the HTTP response body:
-
When the component header includes
json
, the result is parsed into the corresponding object. -
When the component header includes
xml
, the result is converted into the JSON equivalent of the represented XML using the same rules as above. -
When the component header includes
image
,msword
,msexcel
,pdf
,csv
,octet-stream
orbinary
, the request body contents is stored as an attachment. There is nobody
property in the outgoing message. -
When there is no body (because the content-length is 0), there is no
body
property in the outbound message. -
If there is another component, then the response is treated as text.
-
If the component header is omitted, an attempt to convert the result to JSON is made. If that fails, then the result is treated as if it were text.
For more details you can see an example of usage. -
Defining the request’s body
If the HTTP method is anything other than GET
, the Body tab appears next to the Header tab.
The Body tab enables configuration options such as the Component drop-down menu and the Body input field/s.
The following Component values are supported:
-
multipart/form-data
-
application/x-www-form-urlencoded
-
text/plain
-
application/json
-
application/xml
-
text/xml
-
text/html
The Body input field/s change/s according to your chosen Component.
|
Sending JSON data
To send JSON data in the body, change the Component to application/json
.
The Body section changes accordingly to accept a JSON object.
NOTE: This field also supports JSONata expressions.
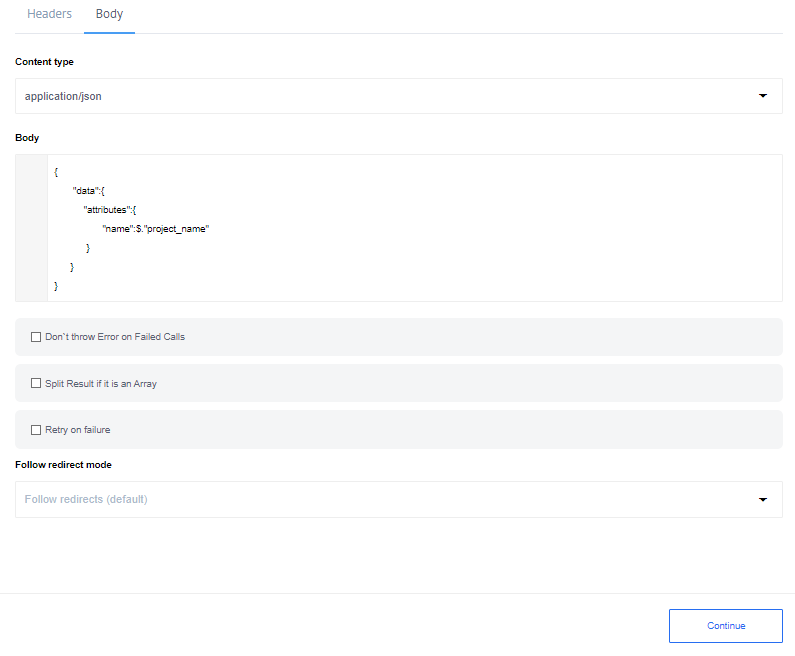
This example shows the JSON in the body where the name
parameter value is mapped using the value of project_name
from the previous integration step.
Sending XML data
To send XML data, set the Component to application/xml
or text/xml
and place the XML in the body input field between double-quotes like:
" <note> <to>" & fname & "</to> <from>Jani</from> <heading>Reminder</heading> <body>Don't forget me this weekend!</body> </note> "
Use a JSONata expression to include and map any values coming from the previous steps.
It replaces the variable with an accurate value in the final mapping.
Note that the rest of XML
gets passed as a string
.
Sending form data
When sending form data, the following content types are available:
-
application/x-www-form-urlencoded
- used to submit simple values to a form. -
multipart/form-data
- used to submit (non-alphanumeric) data or file attachment in payload.
In both cases, the payload gets transmitted in the message body.
For the application/x-www-form-urlencoded
component, add the necessary parameters by giving the name and the values like:
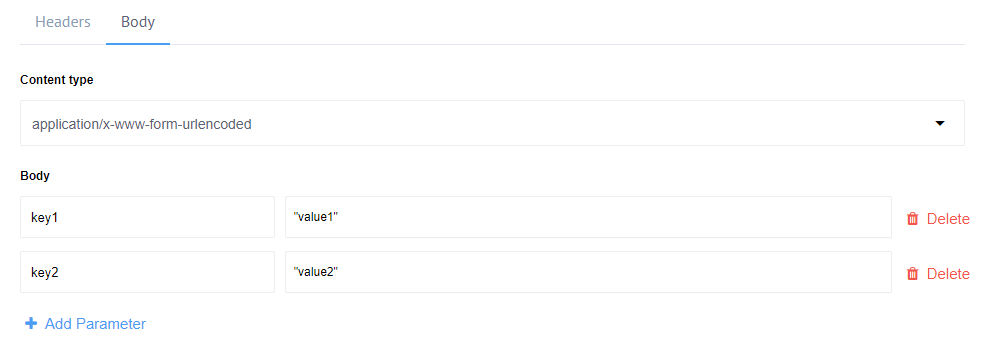
The parameter value fields support JSONata expressions. |
This HTTP request would submit key1=value1&key2=value2
in the message body.
For the multipart/form-data
component, add the parameters similarly.
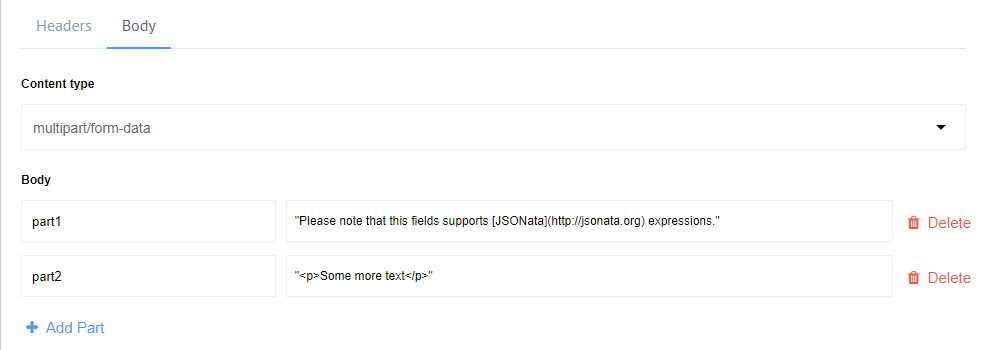
The transmitted HTTP request body would be:
--__X_BOUNDARY__ Content-Disposition: form-data; name="part1" Please note that this field supports [JSONata](http://jsonata.org) expressions. --__X_BOUNDARY__ Content-Disposition: form-data; name="part2" <p>Some more text</p> --__X_BOUNDARY__--
Notice how different parts get separated by the boundary markers. Attachments can also be sent as form data.
Working with XML
The REST API component attempts to parse XML content types in the HTTP response, assuming the Content-Type
header’s MIME Content Type contains the xml
value (e.g. application/xml
).
In such cases, the response body is parsed to JSON using the xml2js
node library, with the following settings:
{
trim: false,
normalize: false,
explicitArray: false,
normalizeTags: false,
attrkey: '_attr',
tagNameProcessors: [
(name) => name.replace(':', '-')
]
}
Learn more about these settings in the XML2JS library 'readme' notes.
HTTP headers
The REST API component allows you to retrieve the HTTP response header only if the Don’t throw Error on Failed Calls option is selected. In such cases, the structure of the output would be:
{
headers:<HTTP headers>,
body:<HTTP response body>,
statusCode:<HTTP response status code>,
statusMessage:<HTTP response status message>
}
Attachments
The REST API component allows you to send binary data.
To do this, set the content type to multipart/form-data
, and attachments from the input message are automatically included in the request payload.
This content type automatically loads binary data to attachments with the next content types in response headers:
-
image/*
-
text/csv
-
application/msword
-
application/msexcgel
-
application/pdf
-
application/octet-stream
-
application/x-binary
-
application/binary
-
application/macbinary
Known limitations
The REST API component has the following limitations:
-
The component can only parse responses whose content types are JSON- or XML-based, for example:
-
application/json
-
application/xml
-
text/xml
If a component is not indicated in the response header, the REST API component attempts to parse the response as JSON. -
If an exception is encountered during parsing, then the response is returned 'as is'.
-
The maximum size for an attachment is 10 MB.
-
The OAuth2 authentication implementation strategy in this component has an access token response limitation, such that the optional
refresh_token
property is required in Integrations. To work around this issue, try using the additional parameteraccess_type:offline
. -
Avoid setting the Delay value to more than the time between two executions of the flow since the delay can influence the time of the subsequent execution. For example, a flow type
Ordinary
and is scheduled to execute every one minute. However, the delay is set to 120 seconds. Therefore the subsequent execution commences only after 120 seconds, instead of one minute.