CSV actions
Read CSV attachment
This action will read the CSV attachment of the incoming message or from the specified URL and output a JSON object.
An example of an object stored in a GitHub repository:
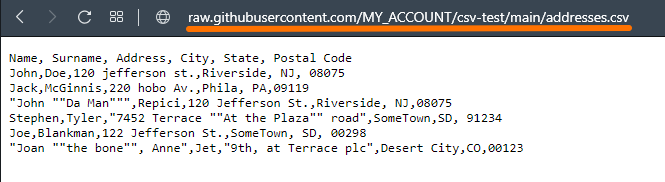
Use the following fields to configure this action:
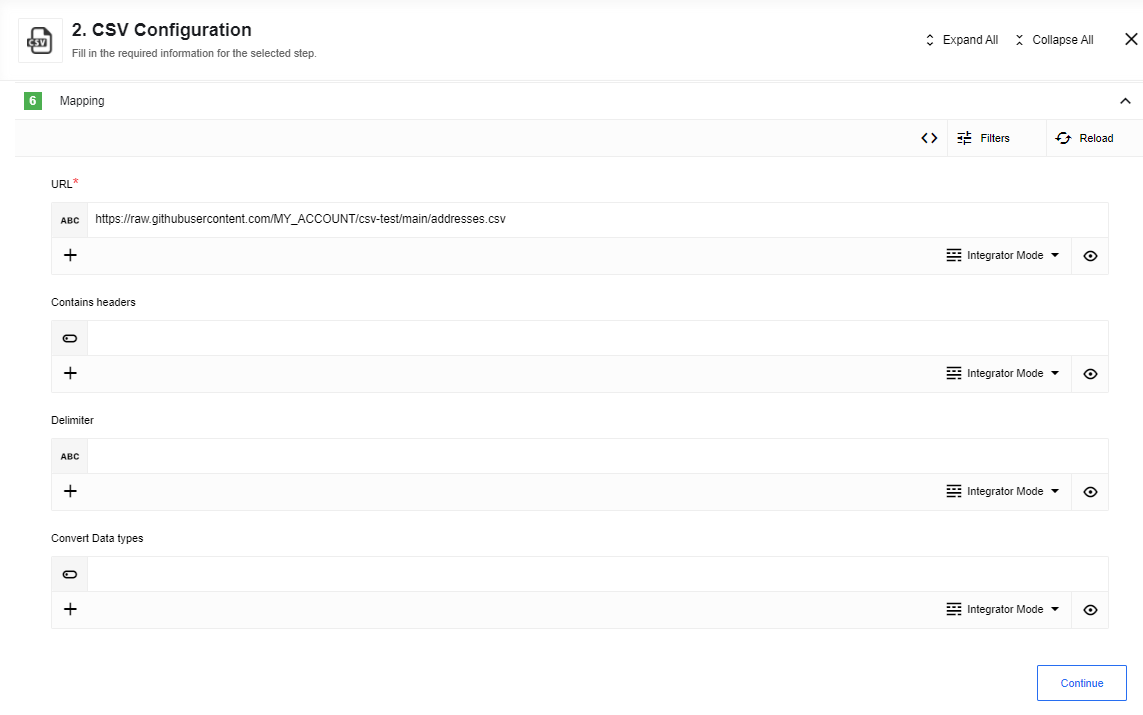
Here you can see an example of a sample:
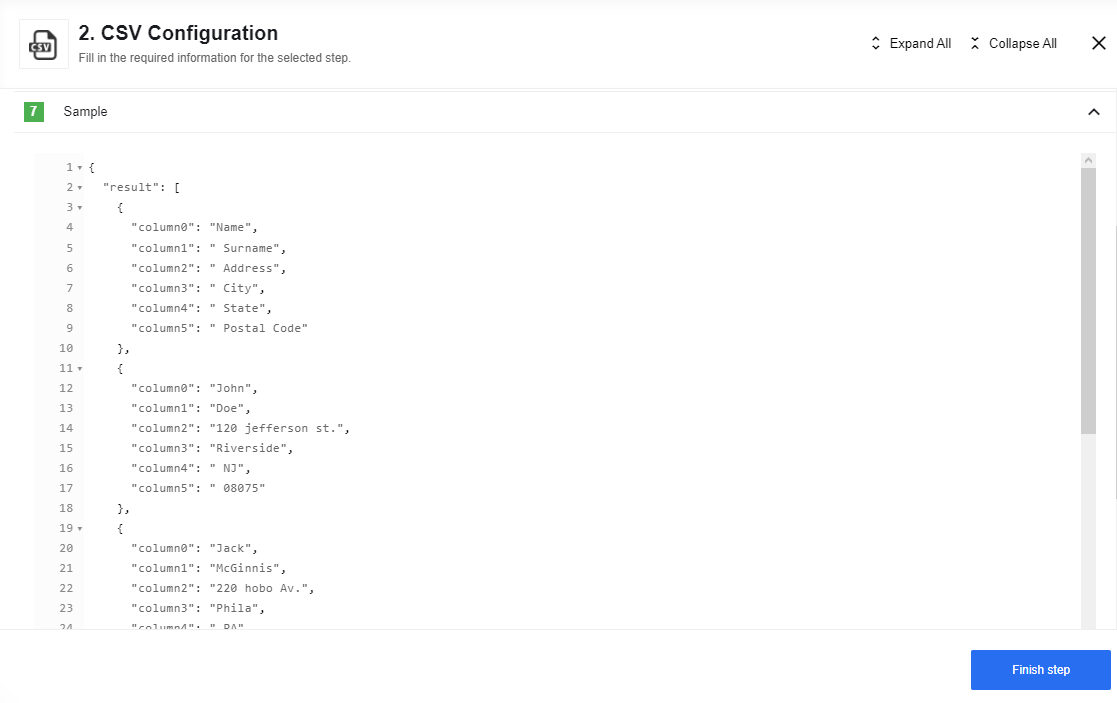
Configuration
- Emit behavior
-
A required drop-down. This selector configures the output behavior of the component.
- Fetch all
-
The component emits an array of messages.
- Emit individually
-
The component emits a message per row.
- Emit batch
-
Component will produce a series of messages. Each message has an array. The maximum length of each array is equal to the Batch size.
- Skip empty lines
-
An optional checkbox. By default, empty lines are parsed. If this checkbox is selected, empty lines will not be parsed. They will be skipped.
- Comment char
-
An optional string. If specified, lines starting with this string will not be parsed. Instead, lines starting with this string will be skipped.
Input metadata
- URL
-
We will fetch this URL and parse it as a CSV file.
- Contains headers
-
If set to
true
, the first row of parsed data will be interpreted as field names. By default this is set tofalse
. - Delimiter
-
The delimiting character. If left blank, the component will auto-detect the delimiting character from a list of the most common delimiters. If a character is added, the added character will be parsed as the delimiting character. Other characters will not. By default this is left blank.
Table 1. Example Delimiter ( $
)JSON a$b$c$d
{ "column0": "a", "column1": "b", "column2": "c", "column3": "d" }
- Convert data types
-
Numeric and boolean data will be converted to their type instead of remaining strings. The default is
false
.
If Emit behavior is set to Emit batch
, a new field appears; Batch size.
This is the maximum length of the array for each message.
Create CSV from message stream
Here you can see a message stream example created using the Node.js component:
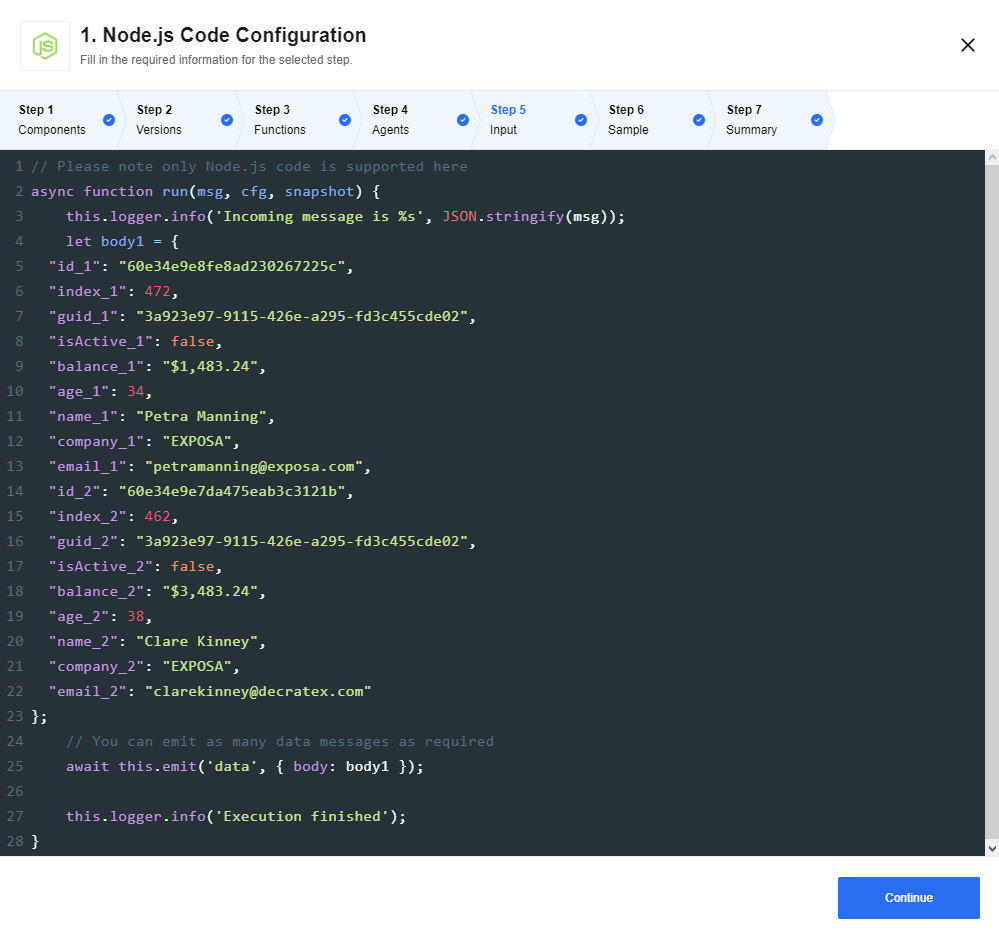
This action combines multiple incoming events into a CSV file until there is a gap of more than 10 seconds between events. Afterward, the CSV file is closed and attached to the outgoing message.
Use the following fields to configure this action:
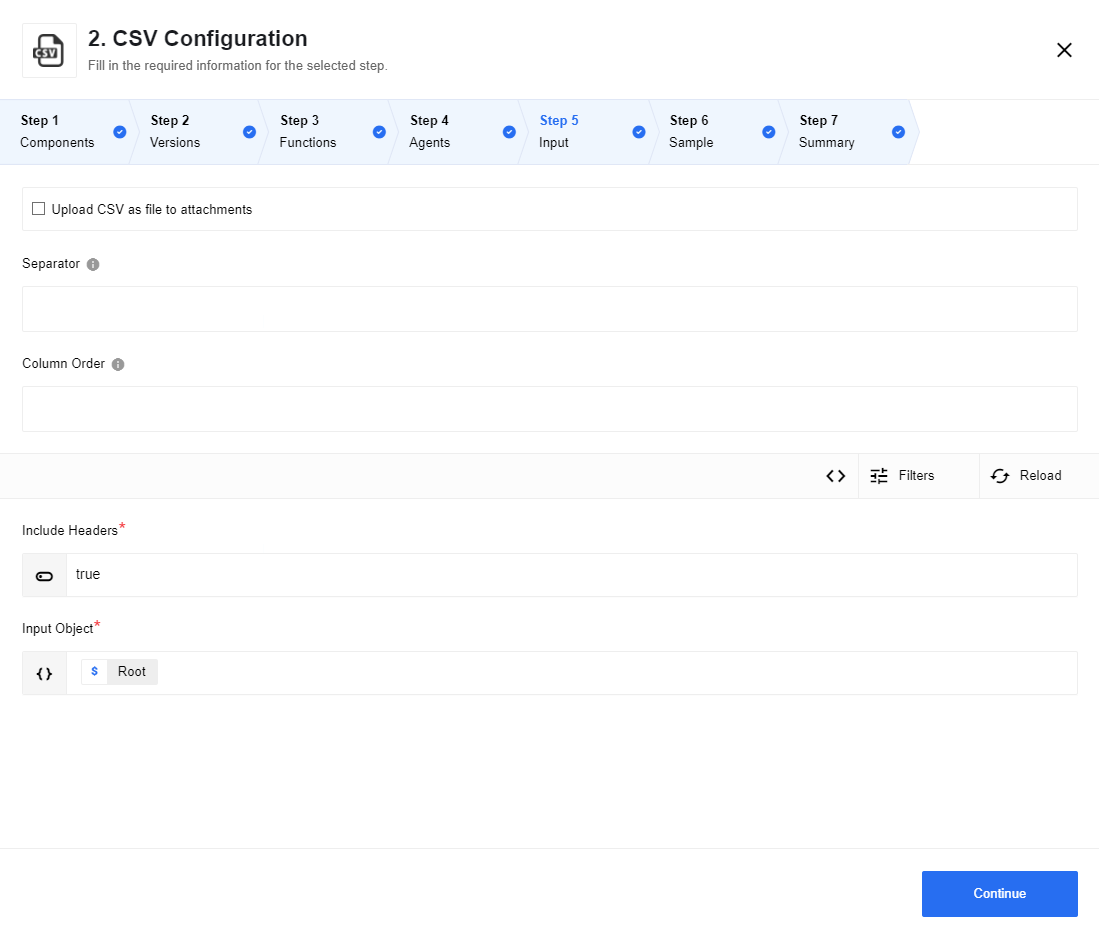
Configuration
- Upload CSV as file to attachments
-
An optional checkbox. If selected, store the generated CSV data as an attachment. If unselected, place the CSV as a string in the outbound message.
- Separator
-
An optional string. A single character that is used to delimit the CSV file. By default this is set to a comma (
,
).The most common delimiters are a comma (
,
), semicolon (;
), tab (\t
), pipe (|
), or space.Any character can be used as a delimiter, however, this can be counterproductive as CSV files are used to pass information between applications and unique delimiters can adversely affect interoperability.
Default ( ,
)Custom ( ;
)Custom ( $
)JSON a,b,c,d
a;b;c;d
a$b$c$d
{ "column0": "a", "column1": "b", "column2": "c", "column3": "d" }
- Column order
-
An optional string. A string, delimited by the separator, defining the columns and in what order they should appear in the resulting file.
If this is omitted, the column order in the resulting file will not be deterministic.
Column names will be trimmed: spaces will be removed from the beginning and end of column names.
Table 2. Example Original Trimmed 'col 1,col 2 ,col 3, col 4'
['col 1', 'col 2', 'col 3', 'col 4']
- New line delimiter
-
An optional string. Defaults to
\r\n
. The character used to determine newline sequence.Other allowed values are set out below.
Table 3. Allowed New line delimiters Value Encoded as New line delimiter default environment CR+LF
\r\n
Windows.
LF
\n
macOS, Linux, BSD, and Unix.
CR
\r
Classic Mac OS and other legacy OSes.
CR+LF
— the default new line delimiter string on Windows — is understood by and parsed as expected by applications that run on Unix and Unix-like operating systems.By contrast,
LF
is not always parsed by Windows applications as expected.CR+LF
is, as a consequence, the sensible default.Only set the new line delimiter to
LF
if a CSV file is only going to be parsed on Unix-like operating systems. - Escape formulae
-
An optional checkbox. If selected, field values that begin with
=
,+
,-
,@
,\t
, or\r
, will be prepended with a ` to defend against injection attacks
Spreadsheet applications, such as Excel and LibreOffice will automatically parse such cells as formulae if this checkbox is left unchecked. |
Input metadata
- Include headers
-
Indicates if a header row should be included in the generated file. This must be a boolean.
- Input object
-
Object to be written as a row in the CSV file. If the Column order is specified, then individual properties can be specified.
Output metadata
- attachmentUrl
-
A URL to the CSV output.
- Type
-
Always set to .csv.
- Size
-
Size in bytes of the resulting CSV file.
- attachmentCreationTime
-
When the attachment was generated.
- attachmentExpiryTime
-
When the attachment is set to expire.
- contentType
-
Always set to text/csv.
- csvString
-
The output CSV as a string inline in the body.
Create CSV from JSON array
This action converts an incoming array into a CSV file. Here you can see a JSON array example created using the Webhook component:
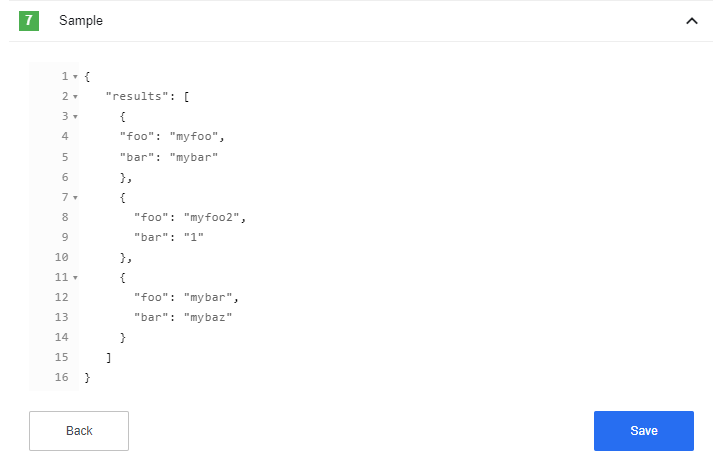
Use the following fields to configure this action:
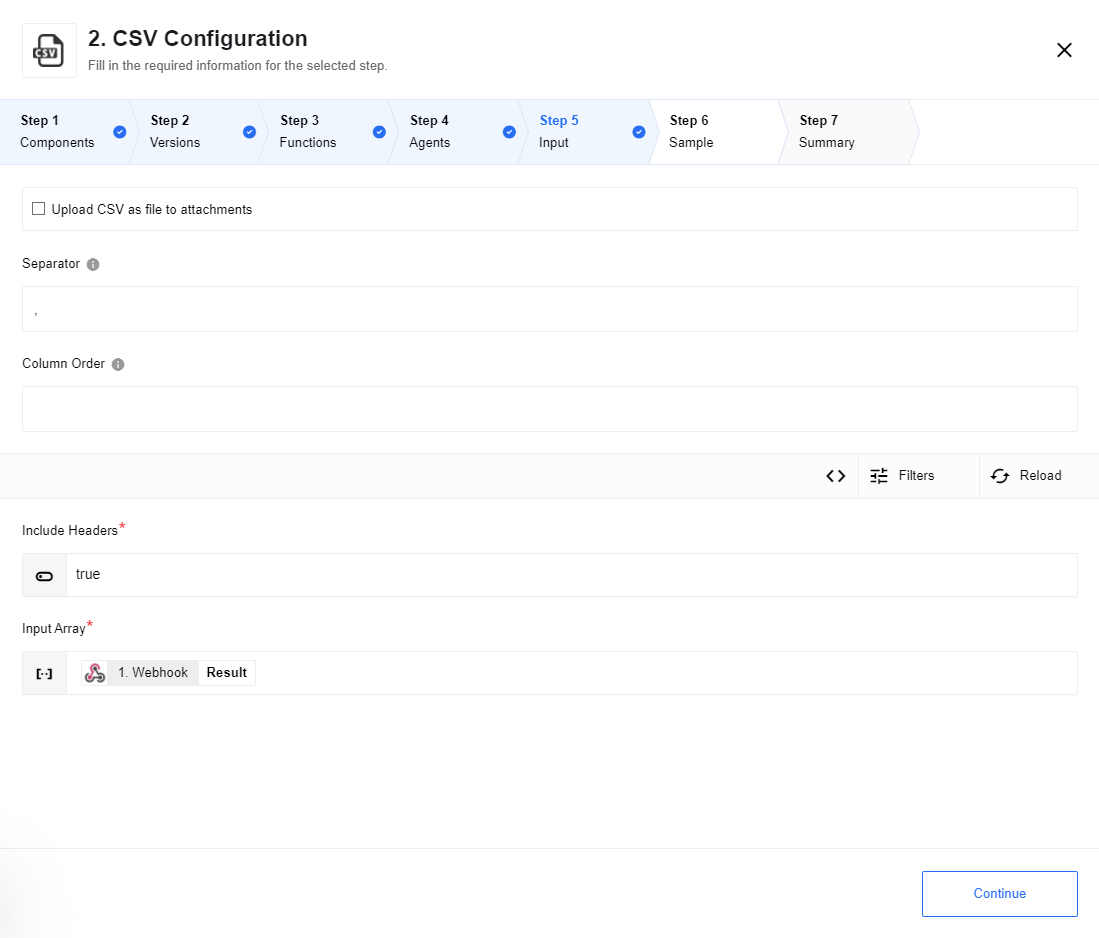
Configuration
- Upload CSV as file to attachments
-
An optional checkbox. If selected, store the generated CSV data as an attachment. If unselected, place the CSV as a string in the outbound message.
- Separator
-
An optional string. A single character that is used to delimit the CSV file. By default this is set to a comma (
,
).The most common delimiters are a comma (
,
), semicolon (;
), tab (\t
), pipe (|
), or space.Any character can be used as a delimiter, however, this can be counterproductive as CSV files are used to pass information between applications and unique delimiters can adversely affect interoperability.
Default ( ,
)Custom ( ;
)Custom ( $
)JSON a,b,c,d
a;b;c;d
a$b$c$d
{ "column0": "a", "column1": "b", "column2": "c", "column3": "d" }
- Column order
-
An optional string. A string, delimited by the separator, defining the columns and in what order they should appear in the resulting file.
If this is omitted, the column order in the resulting file will not be deterministic.
Column names will be trimmed: spaces will be removed from the beginning and end of column names.
Table 4. Example Original Trimmed 'col 1,col 2 ,col 3, col 4'
['col 1', 'col 2', 'col 3', 'col 4']
- New line delimiter
-
An optional string. Defaults to
\r\n
. The character used to determine newline sequence.Other allowed values are set out below.
Table 5. Allowed New line delimiters Value Encoded as New line delimiter default environment CR+LF
\r\n
Windows.
LF
\n
macOS, Linux, BSD, and Unix.
CR
\r
Classic Mac OS and other legacy OSes.
CR+LF
— the default new line delimiter string on Windows — is understood by and parsed as expected by applications that run on Unix and Unix-like operating systems.By contrast,
LF
is not always parsed by Windows applications as expected.CR+LF
is, as a consequence, the sensible default.Only set the new line delimiter to
LF
if a CSV file is only going to be parsed on Unix-like operating systems. - Escape formulae
-
An optional checkbox. If selected, field values that begin with
=
,+
,-
,@
,\t
, or\r
, will be prepended with a ` to defend against injection attacks
Spreadsheet applications, such as Excel and LibreOffice will automatically parse such cells as formulae if this checkbox is left unchecked. |
Input metadata
- Include headers
-
Indicates if a header row should be included in the generated file. This must be a boolean.
- Input array
-
Array of objects to be written as rows in the CSV file (one row per object and headers).
If the Column Order is specified, then individual properties can be specified.
The component will throw an error when the array is empty.
Output metadata
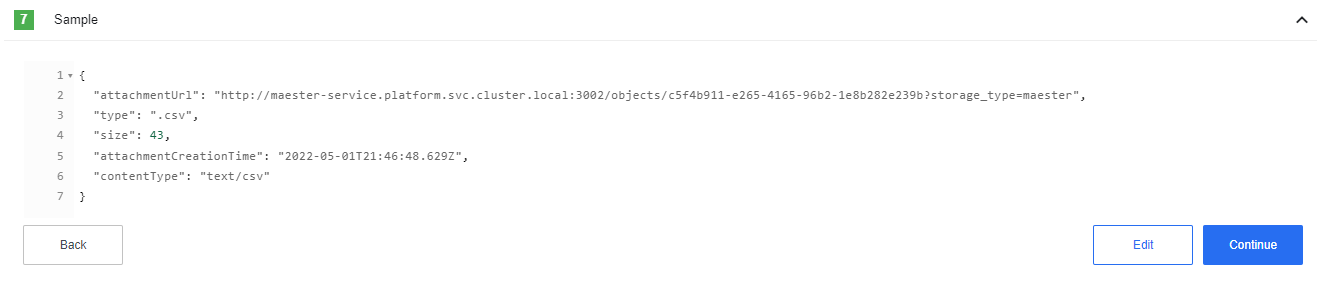
- attachmentUrl
-
A URL to the CSV output.
- Type
-
Always set to
.csv
. - Size
-
Size in bytes of the resulting CSV file.
- attachmentCreationTime
-
When the attachment was generated.
- attachmentExpiryTime
-
When the attachment is set to expire.
- contentType
-
Always set to
text/csv
.

- csvString
-
The output CSV as a string inline in the body.
Limitations
-
You might get the following error during run-time:
Component run out of memory and terminated.
This means that the component needs more memory. Contact the support team for further assistance.
-
The Maximum possible size for an attachment is 10 MB.
-
Inbound message in Message Stream and each element of JSON Array should be a plain
Object
. If the value is not a primitive type, it will be set as[object Object]
.