Node.js code
The Node.js code component lets you run a piece of JavaScript code from within your integration flow.
It has a full-fledged interface with useful features such as:
-
Syntax highlighting.
-
Code auto-completion.
-
Support for several variables and libraries within the context of the execution.
-
Support the latest ECMAScript standard.
-
Run and troubleshoot within the designer interface.
It is deployed by default to production.
Usage
To use the component, start building your integration and include the Node.js code component as well.
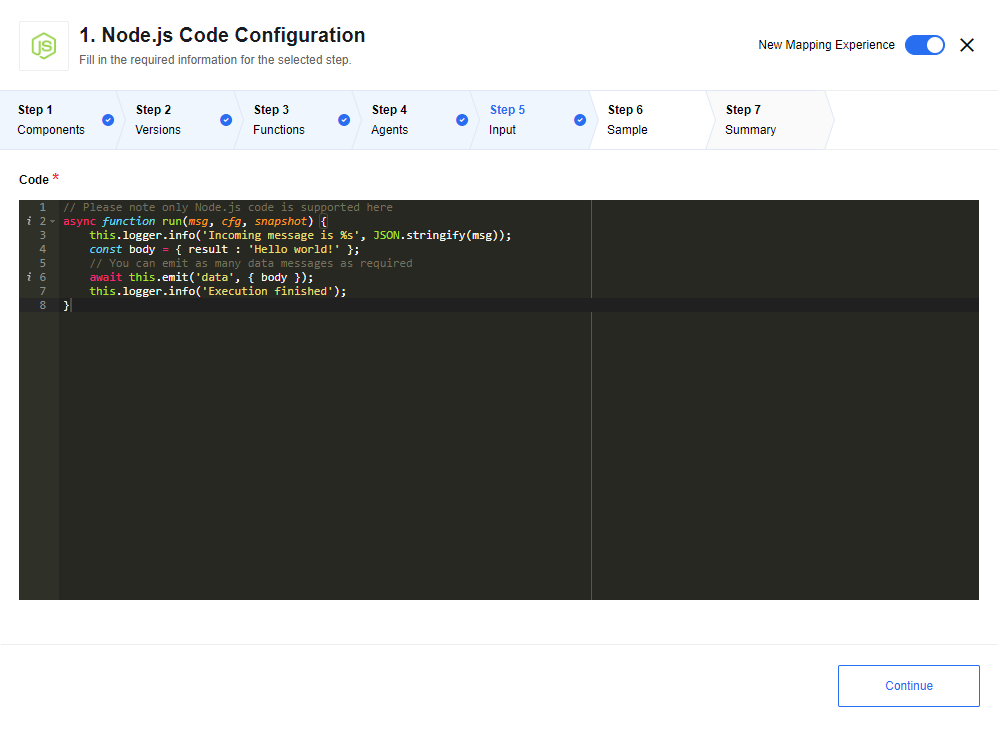
Available variables and libraries
Below is a sample list of the available variables and libraries you can use within the execution context.
The most up-to-date list can always be found within the execution context or in code.js
of the component.
Built-in Node.js global objects are also supported.
Platform specific functionality
- msg
-
The incoming message containing the payload from the previous step.
- cfg
-
The step’s configuration. At the moment contains only one property:
code
(the code being executed). - snapshot
-
The step’s snapshot.
- messages
-
A utility for convenient message creation.
- emitter
-
Used to emit messages and errors.
Other Libraries/functions
- wait(numberOfMilliscondsToSleep)
-
Utility function for sleeping
- request
-
A simplified HTTP client wrapped in co so that it is pre-promisified.
This library has been deprecated. Legacy code can be found at https://github.com/request/request. _
-
Use Lodash to help process arrays, numbers, objects, strings, among other things common in JavaScript
Node.js code component usage examples
To use the code, you can follow these examples:
async function run(msg) {
console.log('Incoming message is %s', JSON.stringify(msg));
const body = { result : 'Hello world!' };
// You can emit as many data messages as required
await this.emit('data', { body });
console.log('Execution finished');
}
async function run(msg, cfg, snapshot) {
return {
addition: 'You can use code',
keys: Object.keys(msg)
};
}
If you have a simple one-in-one-out function, you can return a JSON object resulting from your function. The object is automatically emitted as data. |
Common usage scenarios
Doing complex data transformation
Use the following example to transform an incoming message with code:
async function run(msg) => {
addition : "You can use code",
keys : Object.keys(msg)
}
Calling an external REST API
Use the following example to create a small REST API call from the Node.js code component:
async function run(msg) {
const res = await request.get({
uri: 'http://api.au.connect.squiz.cloud/v2/users', (1)
auth: {
user: process.env.API_USERNAME,
pass: process.env.API_KEY
},
json: true
});
return {
fullName: res.body.first_name + " " + res.body.last_name,
email: res.body.email,
userID: res.body.id
}
}
1 | Read API Documentation for all APIs in supported regions. |