Inline editable
When inline editable is enabled for a component property, it can be edited inline in the preview column.
This allows you to change the text content in the preview column and see the changes as you make them rather than drilling down in the page outline column to make the changes.
The following property types can be configured to be inline editable:
-
string; and
-
SquizImage; and
A dotted line is shown under number
, integer
, and string
fields that can be inline edited.
A dotted rectangle is shown around FormattedText
fields that can be inline edited.
These visible UI markers show editors what components are editable inline and what components must be modified in the page outline column.
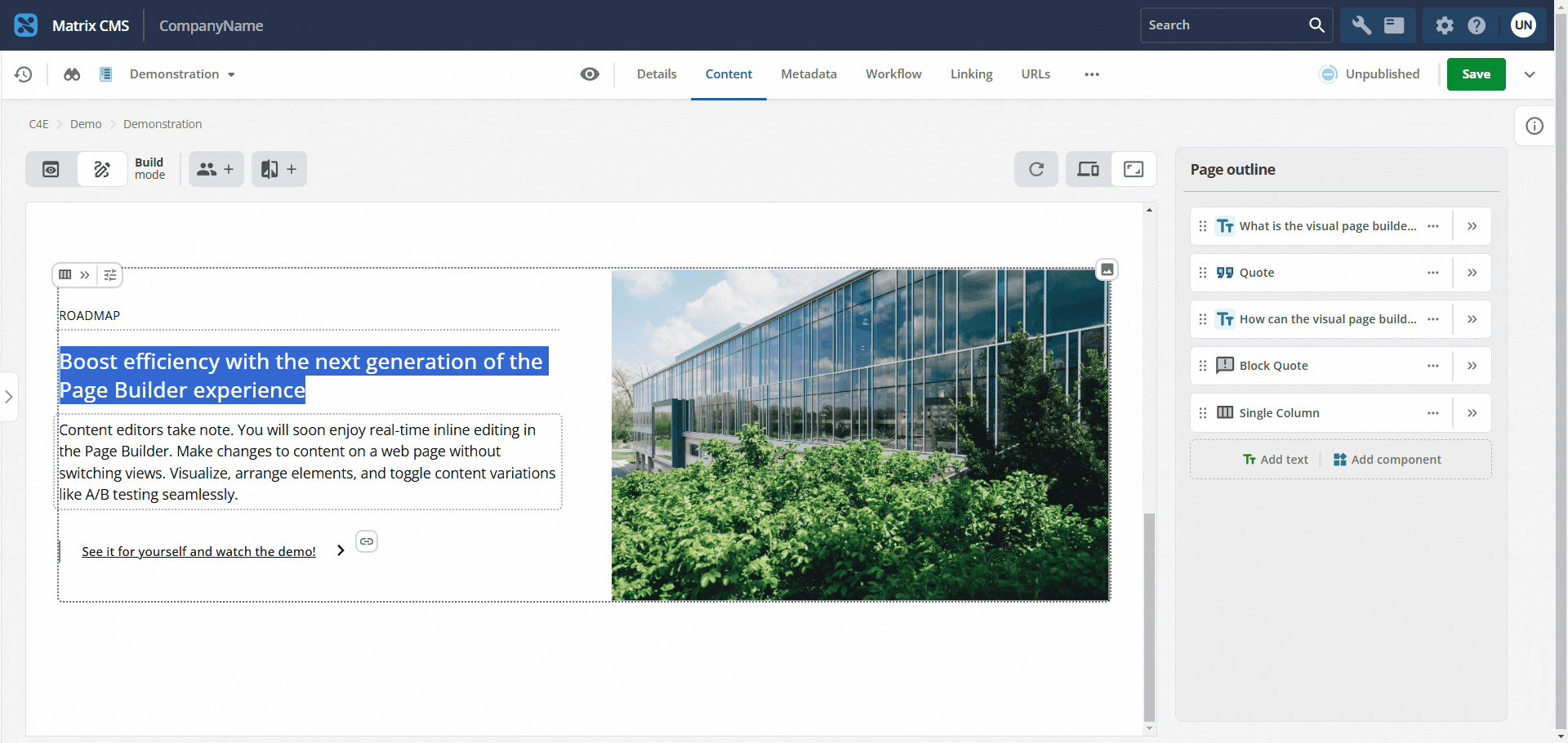
Component changes
Enabling inline editing requires an edit to the manifest.json
file, and an addition to each field in the main.js
file.
ui-metadata
is an optional property that can be added to the component input properties on a manifest.
The values specified on this property inform the component’s interaction behavior in the Visual Page Builder interface.
This property is a non-breaking change that will not affect the component being used on standard pages.
Manifest.json file changes
A simple example:
"ui:metadata": {
"inlineEditable": true
}
A more complete example of a single String property:
"author": {
"type": "string",
"title": "Author",
"ui:metadata": {
"inlineEditable": true
}
}
JavaScript file changes
To make a field inline editable, you must specify these mappings in the main.js
file:
-
The variables passed to the main function.
-
The property defined in the manifest.json file.
This is done by adding a data-sq-field
property to a wrapping HTML element. For example:
<div data-sq-field="author">
${author}
</div>
This sets up a mapping between the passed quote variable, and the quote property defined in the manifest.json
file.
Following is a more complete example: a JavaScript file which outputs a quote and quote author.
As shown, the content — the quoted and the credited author — is not editable inline.
export default {
async main({ quote, author, foregroundColor, backgroundColor }) {
return `<div>
<blockquote>
<div style="color:${foregroundColor}; background-color:${backgroundColor}">
${quote}
</div>
<p>
<em>-- ${author}</em>
</p>
</blockquote>
</div>`;
}
}
To enable inline editing of the quote and author fields, add a data-sq-field
property to a wrapping HTML element. This property maps between the JavaScript parameter and the property defined in manifest.json
.
In this example, add data-sq-field="quote"
to the <div>
tag wrapped around the ${quote}
variable.
For the ${author}
reference there are strings which should not be made inline editable as part of the author field: the <em></em>
tag and the --
text string.
Therefore, add an additional wrapping HTML element, in this case <span></span>
tags, around the ${author}
reference.
export default {
async main({ quote, author, foregroundColor, backgroundColor }) {
return `<div>
<blockquote>
<div data-sq-field="quote" style="color:${foregroundColor}; background-color:${backgroundColor}">
${quote}
</div>
<p>
<em>-- <span data-sq-field="author">${author}</span></em>
</p>
</blockquote>
</div>`;
}
}
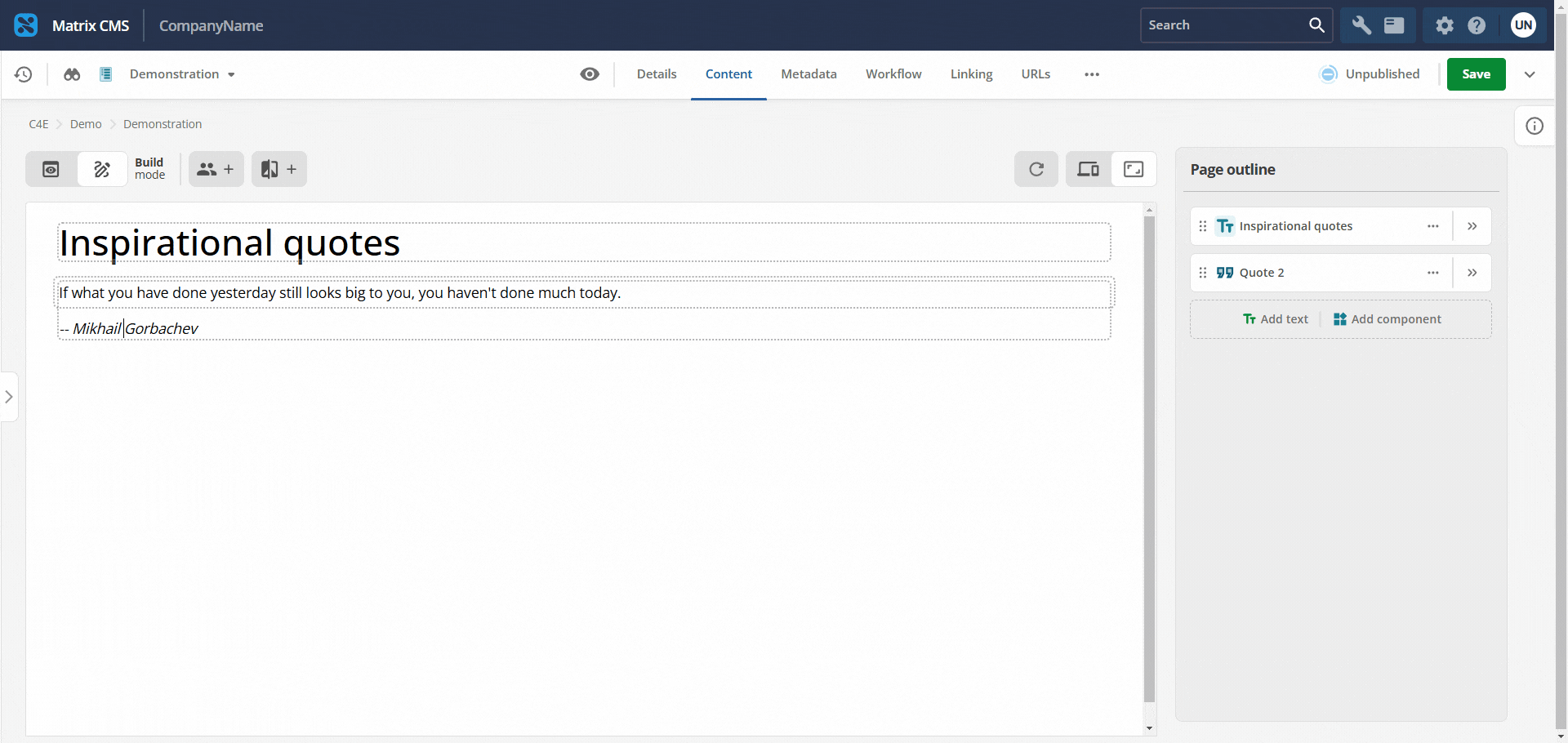
Inline editable allows editors to change text data in the preview column (rather than only in the page outline column).
We can make number number, integer, string, or FormattedText properties available for inline editing.
We can also make SquizImage and SquizLink properties available for inline editing, which we call inline actions.
Inline editing and added complex behaviors
When you build components with inline editing and add complex actions, like event handlers to FormattedText
fields, they will not work in the preview column unless you put a wrapper element around the field.
Attach the event handler to the wrapper instead.
Attaching an event handler to the <div>
around the FormattedText field for a quote will not work.
<div data-sq-field="quote"> (1)
${quote}
</div>
1 | Event handlers attached here will not work. |
We need to add a <div>
wrapper element around the code and attach the event handler to it instead.
<div class="wrapper"> (1)
<div data-sq-field="quote">
${quote}
</div>
</div>
1 | Attach event handlers to this div instead. |