The String input type
One of the simplest yet most powerful input types is that of String. The String type in JSON Schema is used to define properties whose values must be strings.
For more information about the String type in JSON schema, read JSON Schema - String.
Schema definition
To define a property as a string
in JSON Schema, you use the "type" keyword with the value "string".
"input": {
"type": "object",
"properties": {
"textFieldName": {
"title": "example string input",
"type": "string",
"description": "an explanation of what this field is."
}
},
"required": []
},
This example also demonstrates how to provide a description and title to the String field for use within the editing interface.
Basic string constraints
Minimum and maximum length
You can constrain the length of a string using the "minLength" and "maxLength" keywords:
"input": {
"type": "object",
"properties": {
"textFieldName": {
"type": "string",
"minLength": 3,
"maxLength": 50
}
},
"required": []
},
This example ensures that the string has a length between 3 and 50 characters.
Regular expressions
The "pattern" keyword allows you to specify a regular expression pattern that the string must match:
"input": {
"type": "object",
"properties": {
"textFieldName": {
"type": "string",
"pattern": "^[A-Za-z0-9]+$"
}
},
"required": []
},
This example ensures that the string contains only alphanumeric characters.
Format constraints
JSON Schema also provides a "format" keyword for more specific constraints. Some common formats include:
-
"date": String is a date.
-
"email": String is an email address.
-
"uri": String is a URI.
"input": {
"type": "object",
"properties": {
"textFieldName": {
"type": "string",
"format": "email"
}
},
"required": []
},
Multi Line
The Component Service introduces the concept of the multi-line format which transforms the text input into a textarea input within the editing UI.
"input": {
"type": "object",
"properties": {
"textFieldName": {
"type": "string",
"format": "multi-line",
}
},
"required": []
},
Matrix Asset URI
The Component Service introduces the concept of the matrix-asset-uri format, which expects a URI string that is used to resolve a Content API request to a Squiz Content Management System and return an asset object.
"input": {
"type": "object",
"properties": {
"textFieldName": {
"type": "string",
"format": "matrix-asset-uri",
}
},
"required": []
},
The expected value of the matrix-asset-uri
format is in the pattern of matrix-asset://<matrix-api-identifier>/<asset-id>
. For example, a valid value might be matrix-asset://valid-matrix-identifier/1111
where valid-matrix-identifier
is the Matrix API Identifier and 1111
is the asset ID of the Matrix Content Management System asset.
The matrix-asset-uri
value must be resolved using the resolveUri
function of the info.ctx
that is passed to the component execution function. In the following example, the asset 1111
is resolved from the Matrix Content Management System, and the response from the Content Delivery API is printed in a preformatted HTML block.
const input = {
matrixAsset: 'matrix-asset://valid-matrix-identifier/1111'
};
// a component that prints out the asset data response
module.exports = async function (input, info) {
const retrievedMatrixAsset = info.ctx.resolveUri(input.matrixAsset);
return `<pre>${JSON.stringify(retrievedMatrixAsset)}</pre>`;
};
If the URI passed to the info.ctx.resolveUri
function can be resolved successfully, the returned value will be an asset data object in the JSON format.
{
"id": "xxxx",
"type": "page_standard",
"type_name": "Standard Page",
"version": "0.0.1",
"name": "My Folder",
"short_name": "Folder",
"status": {
"id": 2,
"code": "under_construction"
},
"created": {
"date": "2020-02-27T09:47:27+11:00",
"user_id": null
},
"updated": {
"date": "2020-02-27T09:47:27+11:00",
"user_id": ""
},
"published": {
"date": "2020-02-27T09:47:27+11:00",
"user_id": "null"
},
"status_changed": {
"date": "2020-02-27T09:47:27+11:00",
"user_id": "null"
},
"url": "xxx",
"urls": [],
"attributes": {
"test": "test"
},
"additional": {}
}
It’s possible to create a preview mock for local development for matrix-asset-uri
by using the mockedUris
keyword in the manifest file, and creating either an inline, or file based mock object.
"mockedUris": {
"matrix-asset://valid-matrix-identifier/1111": {
"type": "inline",
"value": {
"id": "id-inline",
"type": "root_folder",
"type_name": "Root Folder",
"version": "0.0.1",
"name": "Root Folder",
"short_name": "/",
"status": {
"id": 2,
"code": "under_construction"
},
"created": {
"date": "2020-02-27T09:47:27+11:00",
"user_id": null
},
"updated": {
"date": "2020-02-27T09:47:27+11:00",
"user_id": ""
},
"published": {
"date": "2020-02-27T09:47:27+11:00",
"user_id": "null"
},
"status_changed": {
"date": "2020-02-27T09:47:27+11:00",
"user_id": "null"
},
"url": "xxx",
"urls": [],
"attributes": {
"test": "test"
},
"additional": {}
}
},
"foo://valid-file-uri": {
"type": "file",
"path": "./valid-uri.json"
}
},
In the editing interface, the matrix-asset-uri
format field will be presented as a resource browser which allows users to select assets from the configured Matrix Content Management System, similar to the SquizImage type. See Configure Matrix API Identifier for more information.
Enumerated values
You can specify a list of valid string values using the "enum" keyword:
"input": {
"type": "object",
"properties": {
"textFieldName": {
"type": "string",
"enum": ["red", "green", "blue"]
}
},
"required": []
},
This ensures that the string can only take one of the specified values.
Default value
The "default" keyword allows you to provide a default value for a string property:
"input": {
"type": "object",
"properties": {
"textFieldName": {
"type": "string",
"default": "Hello, World!"
}
},
"required": []
},
Editing interface
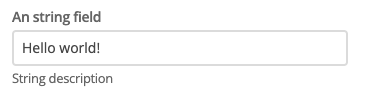
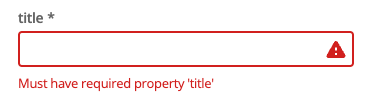
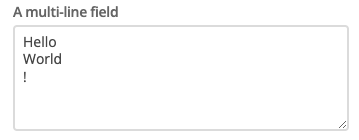
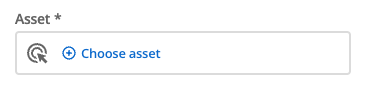
Input object structure
The String input type is available to consume in the main function through the input
object. The String field will output as a basic string variable.
const input = {
stringField: 'the value in the field.'
};
module.exports = async function (input) {
return `
<div>
<p>${input.stringField}</p>
</div>`;
};
Full manifest definition
Expand to see the manifest.json
file with a string input field
{
"$schema": "http://localhost:3000/schemas/v1.json",
"name": "mystring",
"version": "1.0.0",
"mainFunction": "main",
"displayName": "String example",
"namespace": "string-component",
"icon": {
"id": "list_alt",
"color": {
"type": "hex",
"value": "#2D2D2D"
}
},
"description": "This component is set up as a demonstration component for documentation purposes.",
"functions": [
{
"name": "main",
"entry": "main.cjs",
"input": {
"type": "object",
"properties": {
"text": {
"type": "string",
"minLength": 3,
"maxLength": 50
}
},
"required": []
},
"output": { "responseType": "html" }
}
],
"previews":
{
"firstpreview": {
"functionData": {
"main": {
"inputData": {
"type": "inline",
"value": {
"text": "Text supplied to previews function."
}
},
"wrapper": {
"path": "preview-wrapper.html"
}
}
}
}
}
}