Best practices for JavaScript and iFrame implementations inside components
Sometimes, a content page asset’s preview is updated, not the entire content page.
This results in the usual DOM loading events, such as DOMContentLoaded
not being triggered.
Subsequently, any JavaScript whose initialization is based on such events will never retrigger.
Changes are required for components that handle their own JavaScript. These changes also extend to iFrame and other DOM manipulations.
Most of the recommendations in this best practice guide follow the notion of Functional Programming. This approach simplifies the maintenance of scripts built for usage within Page Builder Content Page assets. |
Event Listeners
Event listeners are extensively used and strongly encouraged when additional functionality is required on a page. Read JavaScript HTML DOM EventListener (W3Schools) for more information about event listeners.
These are also recommendations for adding functionality inside of Page Builder including appending an iFrame to the live preview, or to ensure that an action is performed when an accordion or tab is clicked.
As mentioned in the introduction, there are cases where the usual DOMContentLoaded
and script initialization functionality does not work as expected within Page Builder.
Squiz has provided a second event that should be listened for so that functionality can be reinitialized for the live preview.
While this event is livePreviewUpdated
it is important to note that the event is not triggered on the initial page load.
The event only triggers when the preview frame is updated outside of full page load actions, such as when a content editor clicks the Update Preview button on the content page they are editing.
<script>
function initialisation () {
// do amazing things here (1)
furtherActions();
}
function furtherActions () {}
document.addEventListener('DOMContentLoaded', function () {
// content page load actions
initialisation();
});
document.addEventListener('livePreviewUpdated', function () {
// live preview updated only
initialisation();
});
</script>
1 | Read Form embed component for an advanced example of iFrame insertion. |
iFrame recommendations
With the impending deprecation of document.write
Squiz recommends avoiding this approach to place iFrames within a content page webpage.
The recommended approach uses document element creation and appendChild.
Form embed component
The form embed component is recommended for introducing iFrames into a content page asset.
The component is available in your Squiz DXP instance from squiz/edge-form-embed
The component allows for several different implementations to suit each use case.
Building on the example code snippet in the Event Listeners section, observe how the example uses the initialisation
function to append the iFrame to the page preview.
function initialisation () { (1)
var iframe = document.createElement('iframe');
iframe.title="Example iFrame";
iframe.id="an-iframe-example";
iframe.src="url-goes-here";
// either add using formId
document.querySelector('#formid').appendChild(iframe); (2)
// or formClass for multiple instances
document.querySelector('.form-class').appendChild(iframe);
}
1 | The initialisation function appends the iFrame to the page preview. |
2 | The formid property is appended as a child iFrame. |
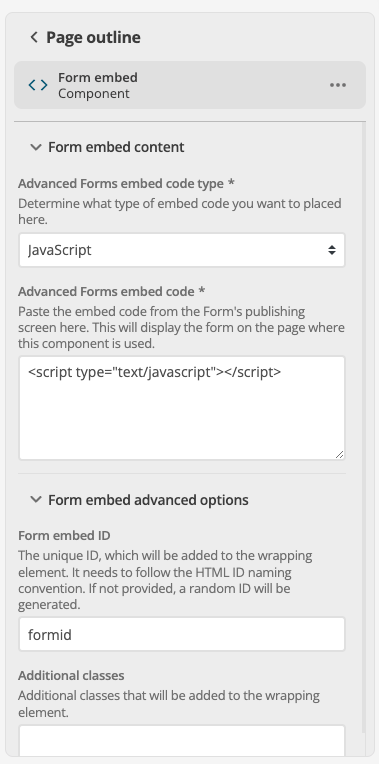