The Boolean input type
In JSON Schema, the Boolean type is used to describe properties that can only have two possible values: true
or false
.
This type is ideal for representing binary decisions or boolean conditions within your JSON data.
For more information about the Boolean type in JSON schema, read JSON Schema - Boolean.
Schema definition
To define a property as a boolean
in JSON Schema, you use the "type" keyword with the value "boolean".
"input": {
"type": "object",
"properties": {
"booleanFieldName": {
"title": "example boolean input",
"type": "boolean",
"description": "an explanation of what this field is."
}
},
"required": []
},
This example also demonstrates how to provide a description and title to the Boolean field for use within the editing interface.
Default value
The "default" keyword allows you to provide a default value for a boolean property:
"input": {
"type": "object",
"properties": {
"boolFieldThatDefaultsTrue": {
"type": "boolean",
"default": true
},
"boolFieldThatDefaultsFalse": {
"type": "boolean",
"default": false
}
},
"required": []
},
Boolean field as a conditional control
The Boolean type can be useful for controlling conditional editing fields within the editing interface.
For example, you may only show an additional String input field for a component when a Boolean input field is set to true
.
This is achieved using the JSON Schema Composition and JSON Schema Conditionals keywords.
"input": {
"type": "object",
"properties": {
"conditionalControlField": {
"default": false,
"title": "Show/hide",
"type": "boolean"
}
},
"allOf": [
{
"if": {
"properties": {
"conditionalControlField": {
"const": false
}
},
"required": []
},
"then": {
"properties": {},
"required": []
}
},
{
"if": {
"properties": {
"conditionalControlField": {
"const": true
}
},
"required": []
},
"then": {
"properties": {
"conditionalResultField": {
"title": "Hidden until shown",
"type": "string"
},
},
"required": ["conditionalResultField"]
}
}
],
"required":[]
},
The |
Editing interface
Boolean toggle field
Use boolean inputs for simple yes/no decisions, enabling/disabling features, or any binary choice in your components.
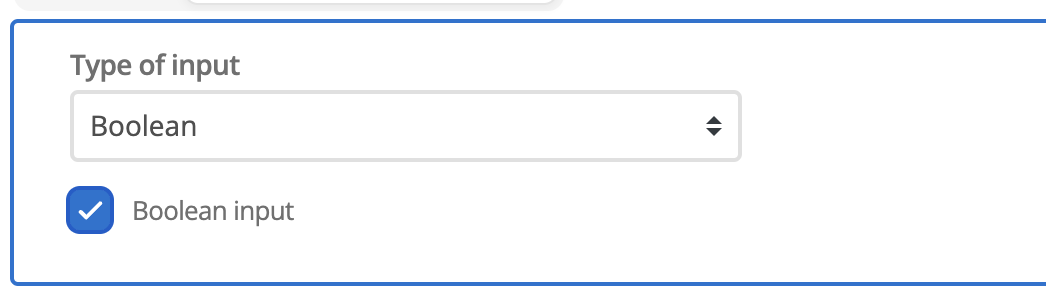
The boolean input appears as a toggle switch that users can click to switch between true (on) and false (off) states. The current state is clearly indicated by the switch position and visual styling.
Input object structure
The Boolean input type is available to consume in the main function through the input
object. The Boolean field will output as either true
or false
only.
const input = {
booleanField: true,
secondBooleanField: false
};
module.exports = async function (input) {
const valueIsTrue = input.booleanField ? "It is true" : "It is false";
return `
<div>
<h1>Is it true?</h1>
<p>${valueIsTrue}</p>
</div>`;
};
Full manifest definition
Expand to see the manifest.json
file with a boolean input field
{
"$schema": "http://localhost:3000/schemas/v1.json",
"name": "mybool",
"version": "1.0.0",
"mainFunction": "main",
"displayName": "Boolean example",
"namespace": "boolean-component",
"icon": {
"id": "list_alt",
"color": {
"type": "hex",
"value": "#2D2D2D"
}
},
"description": "This component is set up as a demonstration component for documentation purposes.",
"functions": [
{
"name": "main",
"entry": "main.cjs",
"input": {
"type": "object",
"properties": {
"boolField": {
"type": "boolean",
"default": false
}
},
"required": []
},
"output": { "responseType": "html" }
}
],
"previews":
{
"firstpreview": {
"functionData": {
"main": {
"inputData": {
"type": "inline",
"value": {
"boolField": true
}
},
"wrapper": {
"path": "preview-wrapper.html"
}
}
}
}
}
}