The Integer input type
In JSON Schema, the integer type is used to describe properties that must have integer values. It allows you to enforce constraints on numeric data, ensuring that values are whole numbers without fractional parts.
For more information about the integer type in JSON schema, read JSON Schema - Integer.
Schema definition
To define a property as a integer
in JSON Schema, you use the "type" keyword with the value "integer".
"input": {
"type": "object",
"properties": {
"IntegerFieldName": {
"title": "example integer input",
"type": "integer",
"description": "an explanation of what this field is."
}
},
"required": []
},
This example also demonstrates how to provide a description and title to the Integer field for use within the editing interface.
Basic integer constraints
Minimum and maximum values
You can constrain the value range of an integer using the "minimum" and "maximum" keywords:
"input": {
"type": "object",
"properties": {
"integerFieldName": {
"type": "integer",
"minimum": 0,
"maximum": 100
}
},
"required": []
},
This example ensures that the integer value falls between 0 and 100 (inclusive).
Exclusive minimum and maximum values
You can constrain the value range of an integer using the "exclusiveMinimum" and "exclusiveMaximum" keywords:
"input": {
"type": "object",
"properties": {
"integerFieldName": {
"type": "integer",
"exclusiveMinimum": 0,
"exclusiveMaximum": 100
}
},
"required": []
},
This example excludes the values 0 and 100, allowing only values between those intervals.
Multiple of
The "multipleOf" keyword allows you to specify that the integer value must be a multiple of a given number:
"input": {
"type": "object",
"properties": {
"integerFieldName": {
"type": "integer",
"multipleOf": 5
}
},
"required": []
},
In this example, the integer must be divisible evenly by 5.
Enumerated values
You can specify a list of valid integer values using the "enum" keyword:
"input": {
"type": "object",
"properties": {
"integerFieldName": {
"type": "integer",
"enum": [42, 7, 11]
}
},
"required": []
},
This ensures that the integer can only take one of the specified values.
Default value
The "default" keyword allows you to provide a default value for an integer property:
"input": {
"type": "object",
"properties": {
"integerFieldName": {
"type": "integer",
"default": 42
}
},
"required": []
},
Editing interface
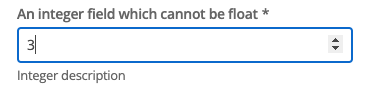
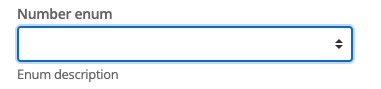
Input object structure
The Integer input type is available to consume in the main function through the input
object. The Integer field will output an integer variable.
const input = {
integerField: 42,
};
module.exports = async function (input) {
return `
<div>
<p><b>Question:</b> How many roads must a man walk down, before you can call him a man?</p>
<p><b>Answer:</b> ${input.integerField}</p>
</div>`;
};
Full manifest definition
Expand to see the manifest.json
file with an integer input field
{
"$schema": "http://localhost:3000/schemas/v1.json",
"name": "myInt",
"version": "1.0.0",
"mainFunction": "main",
"displayName": "Integer example",
"namespace": "integer-component",
"icon": {
"id": "list_alt",
"color": {
"type": "hex",
"value": "#2D2D2D"
}
},
"description": "This component is set up as a demonstration component for documentation purposes.",
"functions": [
{
"name": "main",
"entry": "main.cjs",
"input": {
"type": "object",
"properties": {
"intField": {
"type": "integer",
"default": 42
}
},
"required": []
},
"output": { "responseType": "html" }
}
],
"previews":
{
"firstpreview": {
"functionData": {
"main": {
"inputData": {
"type": "inline",
"value": {
"intField": 42
}
},
"wrapper": {
"path": "preview-wrapper.html"
}
}
}
}
}
}