The Array input type
In JSON Schema, the array type is used to describe an ordered list of items. Arrays allow you to represent collections of values within your JSON data.
For more information about the Object type in JSON schema, read JSON Schema - Array.
Schema definition
To define a property as an array
in JSON Schema, you use the "type" keyword with the value "array".
"input": {
"type": "object",
"properties": {
"exampleArrayField": {
"title": "Example array",
"type": "array",
"items": {
"type": "string"
}
}
},
"required": []
},
This example shows how to create a field representing an array of strings with no fixed length. Similar approaches can be taken to define an array of the other available JSON schema types.
Fixed length arrays
Array types can be configured with a fixed length and with different types for each array item. To enforce a fixed-length array with specific types at each position, use the square bracket array syntax for the "items" keyword.
"input": {
"type": "object",
"properties": {
"exampleArrayField": {
"title": "Example array",
"type": "array",
"items": [
{
"type": "string"
},
{
"type": "number"
},
{
"type": "boolean"
},
{
"type": "string"
},
]
}
},
"required": []
},
This example demonstrates an array field with a length of 4 items, with a string
, number
, boolean
, and then string
type required.
Arrays with constraints
Array types can be configured with constraints to control the minimum and maximum items allowed to exist in the field.
"input": {
"type": "object",
"properties": {
"exampleArrayField": {
"title": "Example array",
"type": "array",
"items": { "type": "string" },
"minItems": 1,
"maxItems": 5
}
},
"required": []
},
This input requires the array to have between 1 and 5 integer items.
Array types can also be configured with a constraint to ensure that all items in the array are unique using the uniqueItems
keyword.
"input": {
"type": "object",
"properties": {
"exampleArrayField": {
"title": "Example array",
"type": "array",
"items": { "type": "string" },
"uniqueItems": true
}
},
"required": []
},
Editing interface
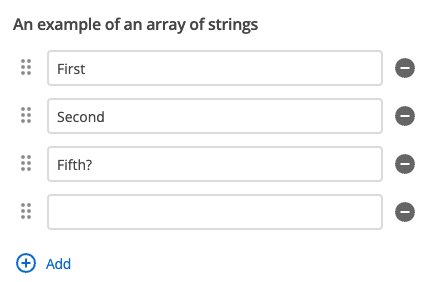
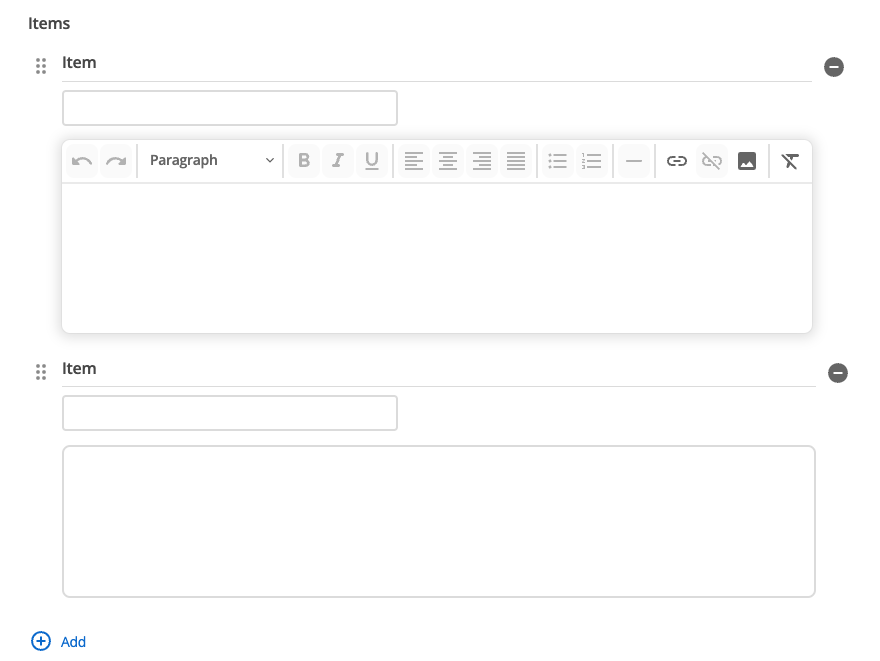
Input object structure
The Array input type is available to consume in the main function through the input
object. As a highly flexible and configurable type, the output structure will depend on the implementation, however it will always be an array at the top level.
const input = {
exampleArrayField: [
"first",
"second",
"fifth"
]
};
module.exports = async function (input) {
return `
<div class="mySquizArrayWrapper">
<ul>
<li>${input.exampleArrayField[0]}</li>
<li>${input.exampleArrayField[1]}</li>
<li>${input.exampleArrayField[2]}</li>
</ul>
</div>`;
};
Full manifest definition
Expand to see the manifest.json
file with a string input field
{
"$schema": "http://localhost:3000/schemas/v1.json",
"name": "myObject",
"version": "1.0.0",
"mainFunction": "main",
"displayName": "Array example",
"namespace": "array-component",
"icon": {
"id": "list_alt",
"color": {
"type": "hex",
"value": "#2D2D2D"
}
},
"description": "This component is set up as a demonstration component for documentation purposes.",
"functions": [
{
"name": "main",
"entry": "main.cjs",
"input": {
"type": "object",
"properties": {
"exampleArrayField": {
"title": "Example array",
"type": "array",
"description": "An array of strings",
"items": { "type": "string" },
}
},
"required": []
},
"output": { "responseType": "html" }
}
],
"previews": {
"firstPreview": {
"functionData": {
"main": {
"inputData": {
"type": "inline",
"value": {
"exampleArrayField": ["first", "second", "fifth"]
}
}
}
}
}
}
}